Dynamic Programming
Introduction
Dynamic Programming (DP) is a powerful optimization technique used in computer science and mathematics to solve problems that can be broken down into overlapping subproblems. Unlike naive recursive approaches, dynamic programming efficiently solves problems by solving each subproblem only once and storing the results for future reference. This essay explores the principles, applications, and advantages of dynamic programming, shedding light on its significance in algorithm design.
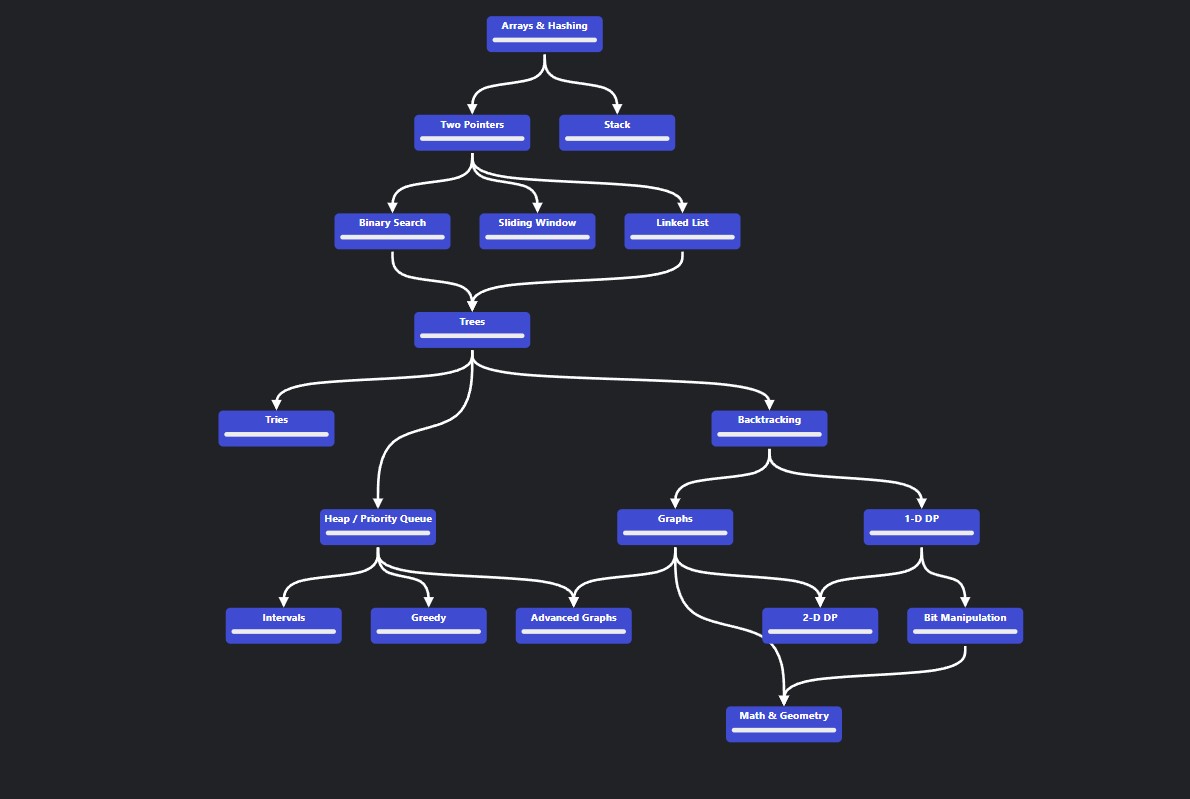
Introduction videos
Fundamental Principles:
At the core of dynamic programming lies the principle of optimal substructure and overlapping subproblems. Optimal substructure implies that an optimal solution to a problem can be constructed from optimal solutions to its subproblems. Overlapping subproblems occur when the same subproblems are solved multiple times in the course of solving the overall problem. Dynamic programming addresses this inefficiency by storing the solutions to subproblems in a table, ensuring that each subproblem is solved only once.
Memoization vs. Tabulation:
Dynamic programming can be implemented using two main approaches: memoization and tabulation. Memoization involves storing the results of subproblems in a data structure (usually a hash table) to avoid redundant computations during recursive calls. Tabulation, on the other hand, involves building a table and filling it iteratively, starting from the smallest subproblems and progressing to the overall problem. Both approaches have their merits, and the choice between them often depends on the problem at hand and the available resources.
Advantages of Dynamic Programming
Optimal Solutions
Dynamic programming guarantees optimal solutions to subproblems, leading to an optimal solution for the overall problem.
Efficiency
By avoiding redundant computations through the use of memoization or tabulation, dynamic programming significantly improves the efficiency of algorithmic solutions.
Versatility
Dynamic programming is a versatile technique applicable to a wide range of problems, from numerical optimization to combinatorial challenges.
Simplicity
While some problems may initially seem complex, dynamic programming allows for breaking them down into simpler, manageable subproblems, making them more tractable.
MIT Lecture: Dynamic Programming I: Fibonacci, Shortest Paths
Dynamic Programming - Learn to Solve Algorithmic Problems & Coding Challenges (5 hours course)
Dynamic Programming 1D - Python (3 hours course)
Dynamic Programming 2D - Python (3 hours course)
Graph Algorithms
Introduction
Graph algorithms are a set of computational procedures designed to analyze and manipulate graphs, which are mathematical structures consisting of nodes (vertices) and edges. These algorithms are fundamental in computer science, as graphs model relationships and connections between entities in various real-world and theoretical scenarios. Here, I'll explain some key graph algorithms and their applications.
Graph Algorithms (2 hours course)
Advantages of Dynamic Programming
Breadth-First Search (BFS)
BFS explores a graph level by level, visiting all neighbors of a node before moving on to the next level.
Depth-First Search (DFS)
DFS explores a graph by going as deep as possible along each branch before backtracking.
Dijkstra's Algorithm
Dijkstra's algorithm finds the shortest path from a source node to all other nodes in a weighted graph.
Bellman-Ford Algorithm
Bellman-Ford finds the shortest paths in a weighted graph, even when it contains negative weight edges (unlike Dijkstra's algorithm).
Minimum Spanning Tree (Prim's and Kruskal's Algorithms)
Find the minimum spanning tree (MST) of a graph, a tree that spans all nodes with the minimum possible total edge weight.
Floyd-Warshall Algorithm
Floyd-Warshall calculates the shortest paths between all pairs of nodes in a weighted graph.
Topological Sorting
Arrange the nodes of a directed acyclic graph (DAG) in a linear order respecting the partial order given by the edges.
Maximum Flow (Ford-Fulkerson Algorithm)
Find the maximum flow in a network, representing the maximum amount of flow that can be sent from a source to a sink.
Graph Coloring
Assign colors to the nodes of a graph in such a way that no two adjacent nodes share the same color.